- Published on
How to Avoid Making Your GraphQL API a REST-QL API
- Authors
- Name
- Andrew Blase
Summary
In the rapidly evolving landscape of API development, GraphQL has emerged as a powerful alternative to traditional REST APIs. However, for software engineers transitioning from REST to GraphQL, understanding the underlying principles of graph theory becomes crucial. In this article, we will explore how graph theory plays a pivotal role in building robust GraphQL APIs and optimizing query resolution. Along the way, we'll delve into real-world experiences, examine practical implications, and discover how a simple change in resolving subtypes transformed a company's GraphQL API, leading to cleaner code and more efficient queries.
Introduction
Imagine a world where developers can effortlessly fetch precisely the data they need, avoiding the pitfalls of over-fetching or under-fetching. Enter GraphQL, a revolutionary query language and runtime that empowers developers to mold APIs according to their data needs. However, to truly harness the power of GraphQL, developers must embark on a journey into the fascinating realm of graph theory. In this article, we will uncover the untapped potential of graph theory in GraphQL API development and witness its transformative impact on real-world scenarios.
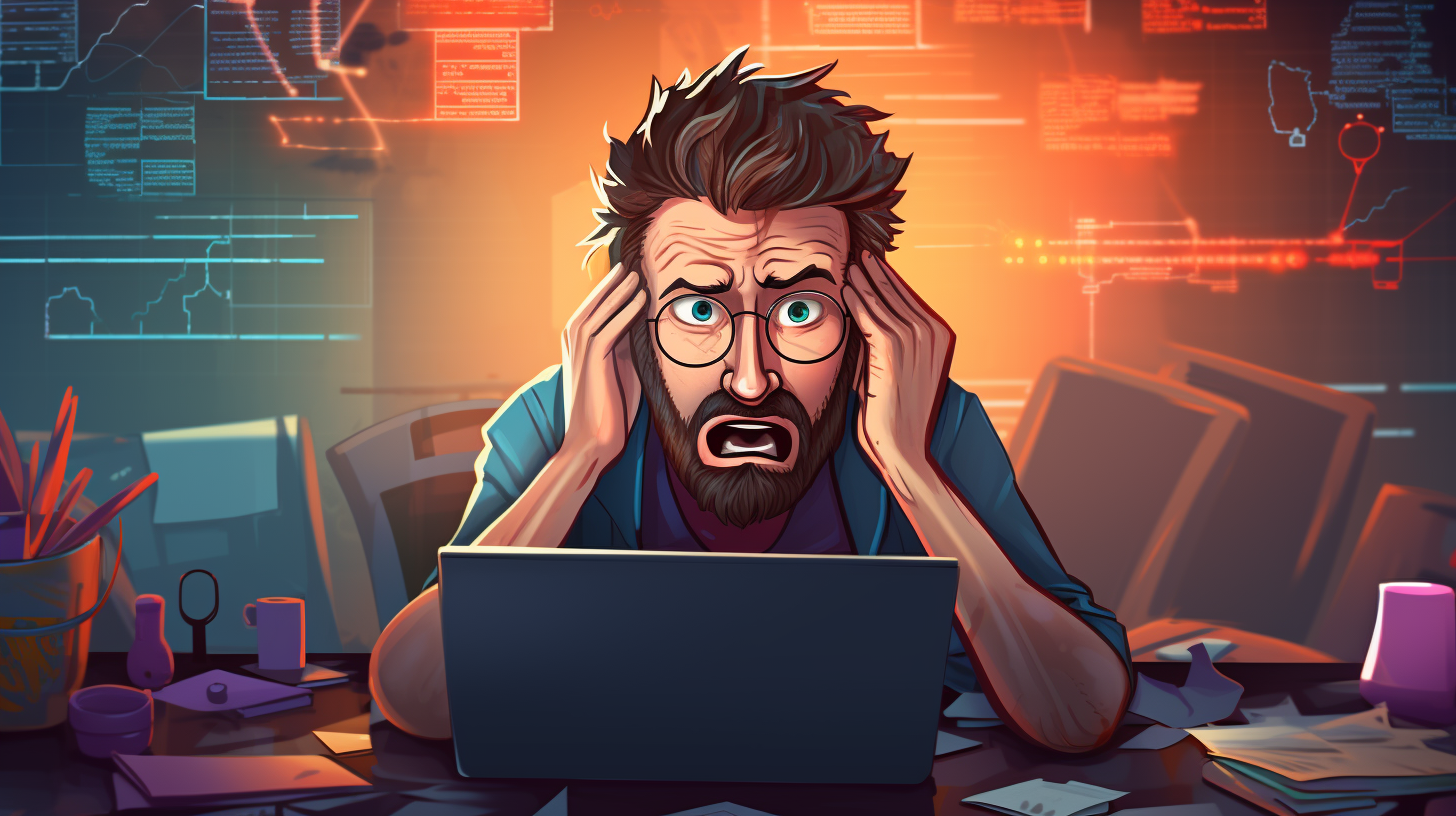
Understanding Graph Theory
At its core, graph theory provides a powerful foundation for understanding relationships between entities. Nodes, edges, and directed acyclic graphs (DAGs) form the building blocks of graph theory, and these concepts are essential in comprehending the inner workings of GraphQL APIs. Let's take a moment to dive deeper into these fundamental concepts and explore how they intersect with GraphQL.
Graph Theory: An Introduction
Graph theory is a branch of mathematics that studies the relationships between objects, represented as nodes or vertices, and the connections between them, represented as edges. It provides a framework for analyzing and understanding complex networks, such as social networks, computer networks, and data relationships.
Nodes, Edges, and Directed Acyclic Graphs (DAGs)
In graph theory, nodes represent entities or data points, while edges represent the relationships or connections between these entities. Directed acyclic graphs (DAGs) are a particular type of graph that do not contain cycles, meaning there are no loops or circular dependencies. DAGs are relevant in the context of graph theory and GraphQL because they represent a structure that can be efficiently traversed and queried.
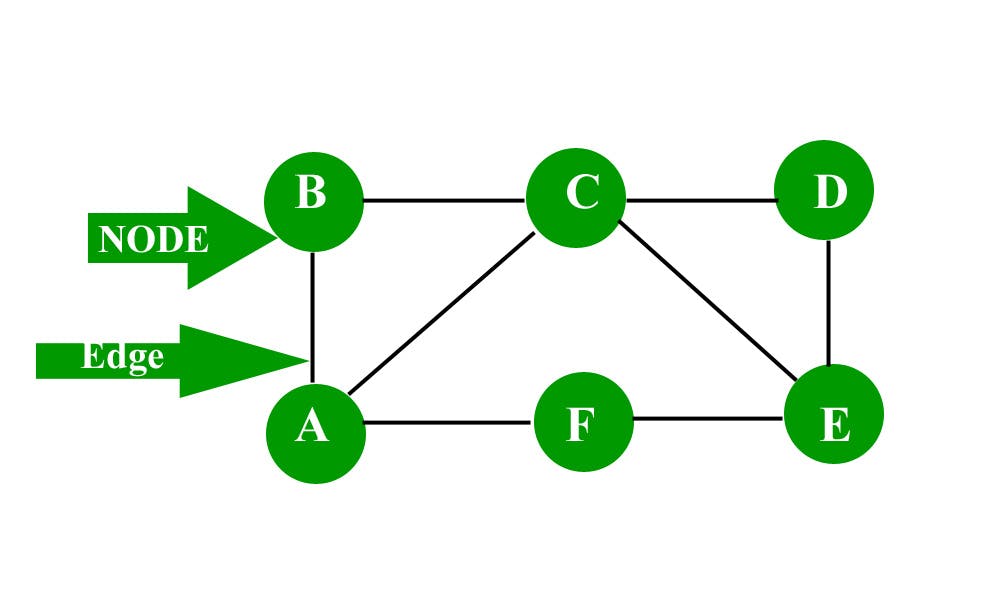
Graph Theory and GraphQL
When GraphQL emerged, it not only introduced a new query language but also embraced the principles of graph theory. By leveraging these principles, GraphQL represents data as a graph, connecting various data types and enabling seamless resolution of relationships. This graph-like structure empowers developers to design APIs that are more flexible, scalable, and optimized for efficient data retrieval.
Expanding on the Benefits of GraphQL
Before delving further into graph theory's role, let's explore the advantages of GraphQL over traditional REST APIs. By embracing GraphQL, developers can bid farewell to the era of over-fetching or under-fetching data. GraphQL's elegant schema and type system allow clients to precisely request the data they need, eliminating unnecessary network requests and reducing data transfer overhead. This fine-grained control enables faster development, improved client experiences, and more efficient use of resources.
Optimizing Query Resolution: A Real-World Story:
Let's venture into a real-world story that vividly demonstrates the impact of graph theory on GraphQL API development. In a recent project, a company embarked on their first GraphQL API implementation. Initially, they attempted to resolve all queries within the actual query itself, including the resolution of subtypes. However, this approach led to an explosion of code for each query and arduous data transformations on the returned data.
Recognizing the potential of graph theory, an experienced engineer intervened and introduced a critical change. Instead of resolving subtypes within the query, they shifted the responsibility to the types themselves. This seemingly minor adjustment unleashed a wave of transformation. Code became cleaner, more self-contained, and easily maintainable. Moreover, this change enabled the underlying ORM (Object-Relational Mapping) to construct more efficient queries, leading to significant performance improvements.
Simplified example of how the original code looked:
const resolvers = {
Query: {
user(parent, args, contextValue, info) {
const user = await prisma.user.findUnique({
where: {
email: args.where.email,
},
});
const products = await prisma.product.findMany({
where: {
userId: user.id,
},
});
const companies = await prisma.company.findMany({
where: {
and: products.map...,
},
});
...
return ...
},
},
};
Code that uses the Graph: There is a more efficient way of doing this with a code-first approach, which is covered in this article:
const resolvers = {
Query: {
user(parent, args, context, info) {
return await context.prisma.user.findUnique({
where: {
email: args.where.email,
},
});
},
},
user: {
products(parent, args, context, info) {
return await prisma.product.findMany({
where: {
user: { id: parent.userId },
},
});
},
},
product: {
companyID: string,
company(parent, args, context, info) {
return await prisma.company.findOne({
where: {
id: parent.companyID,
},
});
},
},
};
This will be much more efficient as a query to get products will automatically resolve all companies no matter the parent of the products.
Practical Implications: Leveraging Graph Theory in GraphQL API Development
The real-world story highlights just one instance where graph theory guided the transformation of a GraphQL API. However, the practical implications extend far beyond. By understanding the fundamental principles of graph theory, developers can make informed decisions in GraphQL API design, optimize query resolution, and build more efficient and maintainable code.
Consider a scenario where a social media platform utilizes GraphQL to handle complex data relationships between users, posts, and comments. By leveraging graph theory, developers can model these relationships as nodes and edges, facilitating efficient traversal and retrieval of related data. This approach enhances the platform's performance and scalability while providing a seamless user experience.
Furthermore, graph theory enables the identification of patterns and the application of powerful graph algorithms within GraphQL APIs. Algorithms like depth-first search (DFS) or breadth-first search (BFS) can be utilized to traverse the graph and extract relevant data efficiently. Topological sorting can assist in handling dependencies and sequencing operations. These graph algorithms empower developers to optimize data fetching, enabling faster response times and reducing unnecessary computational overhead.
The Relay Spec: Bridging Graph Theory and GraphQL
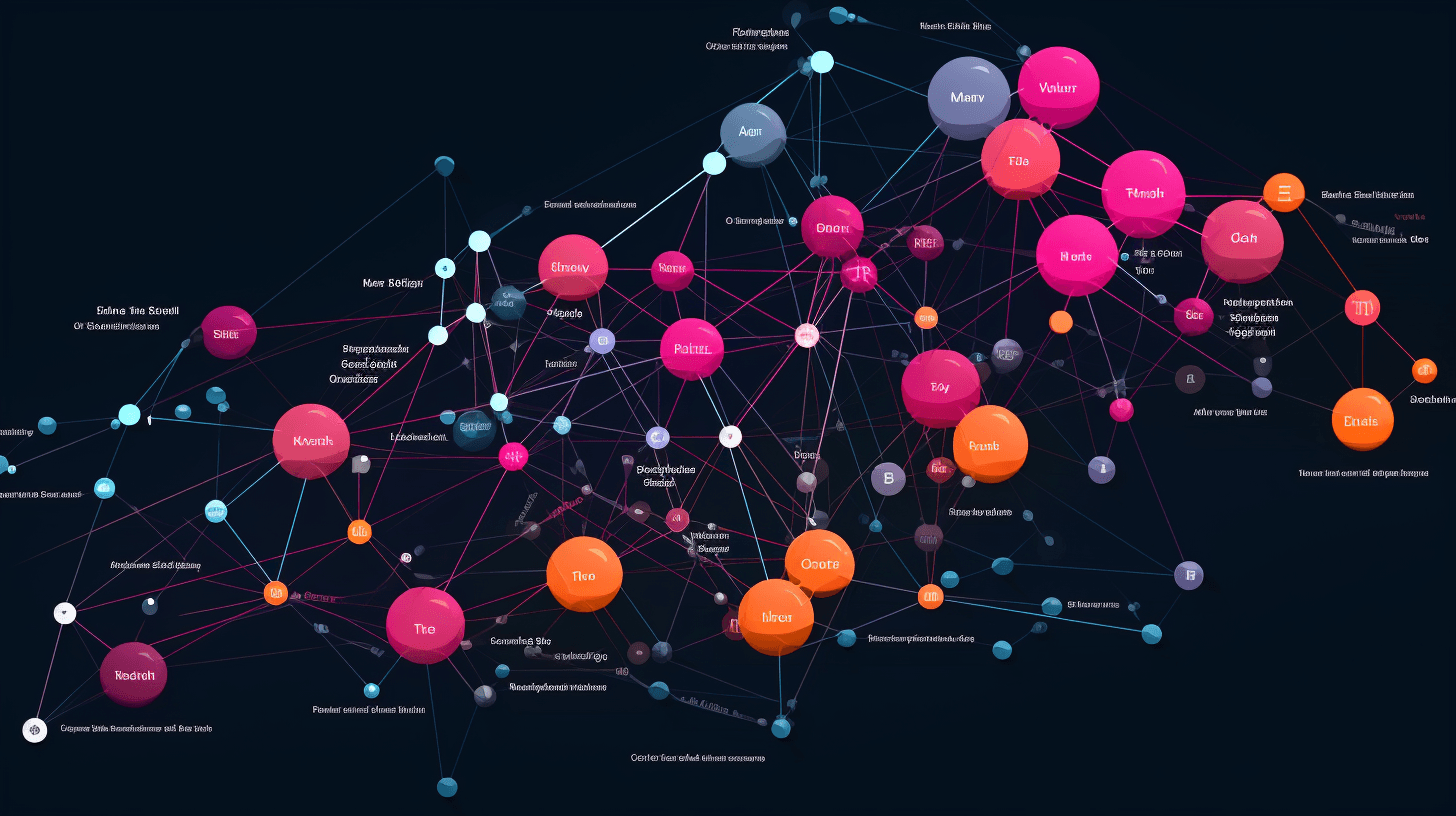
Another significant aspect of GraphQL that intersects with graph theory is the Relay specification. Relay provides guidelines for structuring GraphQL APIs, emphasizing concepts such as edges and nodes. Understanding how the Relay spec relates to graph theory empowers developers to build clean and efficient GraphQL APIs that adhere to industry standards. By adhering to the Relay spec, developers can design APIs that are not only performant but also seamlessly integrate with Relay-powered client frameworks.
Conclusion
In the journey from REST to GraphQL, software engineers must embrace the principles of graph theory to fully unlock the power of GraphQL APIs. By incorporating graph theory concepts, developers can design APIs that leverage the inherent graph-like nature of GraphQL. The real-world story of the transformative impact of resolving subtypes showcases the practical implications of graph theory on GraphQL API development.
By bridging the gap between graph theory and GraphQL, developers revolutionize how data is accessed, manipulated, and consumed, empowering them to build transformative applications in a data-centric world. So, let's embark on this journey together, where graph theory and GraphQL converge, to shape the future of API development.
Resources for Further Learning:
- Relay Specification Documentation
- "The Modern GraphQL Bootcamp" (Udemy course by Andrew Mead)
- "GraphQL: Understanding the Basics" (YouTube video by Fireship)
- "Graph Theory and Its Applications" (Book by Jonathan L. Gross and Jay Yellen)
- "Learning GraphQL: Declarative Data Fetching for Modern Web Applications" (Book by Eve Porcello and Alex Banks)
Learn how to get twice as much done with a fifth of the code.